本文代码基于 Swift5 。
什么情况下我们需要设置 label 的内边距呢?
需要设置的情况:按字数自适应宽度的 label,并且还要有一定的边距。
如下图:标签的长度是按照字数自适应的,且左右要保持 10 pt 的间距。
代码解决方案
|
|
使用
|
|
代码解读
首先创建一个 UILabel 的子类 InsetLabel ,在类里面定义一个接受间距的属性: textInsets。然后通过重写 textRect(forBounds:limitedToNumberOfLines:)
方法来返回添加了间距的正确 bounds。
最后,通过重写 drawTextInRect:
方法,用正确的 bounds 来绘制文本内容。
textRect(forBounds:limitedToNumberOfLines:)
:在系统执行其他文本布局计算之前,在子类重写该方法来实现需要的 label bounds。drawTextInRect:
:重写该方法来配置当前的上下文,然后调用父类的方法来进行真正的绘制。支持IB
1234567891011121314151617181920212223242526@IBDesignableextension InsetLabel {@IBInspectablevar leftTextInset: CGFloat {set { textInsets.left = newValue }get { return textInsets.left}}@IBInspectablevar rightTextInset: CGFloat {set { textInsets.right = newValue }get { return textInsets.right}}@IBInspectablevar topTextInset: CGFloat {set { textInsets.top = newValue }get { return textInsets.top}}@IBInspectablevar bottomTextInset: CGFloat {set { textInsets.bottom = newValue }get { return textInsets.bottom}}}
使用
直接设置 IB 中 Inspectors 栏中的属性值即可。
效果
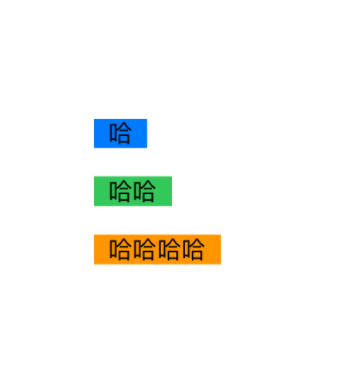